Key Takeaways
Integrating GitHub Actions with Kubernetes simplifies the continuous deployment process, making it more efficient and reliable.
Setting up a GitHub repository and a Kubernetes cluster are essential first steps in creating a seamless CI/CD pipeline.
GitHub Actions automates workflows for building, testing, and deploying containerized applications.
Managing secrets and environment variables is crucial for secure and effective deployment.
Continuous monitoring and handling failures are key to maintaining a robust CI/CD pipeline.
Streamlining DevOps: Integrating GitHub Actions with Kubernetes for Continuous Deployment
Why Integrate GitHub Actions and Kubernetes?
Integrating GitHub Actions with Kubernetes is a game-changer for developers looking to streamline their DevOps processes. GitHub Actions allows you to automate workflows directly from your GitHub repository. This means you can set up actions to build, test, and deploy your code whenever you push changes. On the other hand, Kubernetes excels at managing containerized applications at scale. Combining these two powerful tools can simplify your continuous deployment process, making it more efficient and reliable.
Most importantly, this integration can help reduce the manual steps involved in deploying applications. By automating these steps, you minimize the risk of human error and ensure a consistent deployment process. This is particularly beneficial for teams working in agile environments where frequent updates and quick iterations are the norm.
Benefits of Automated Continuous Deployment
Automated continuous deployment offers several advantages:
Consistency: Automated deployments ensure that every release is deployed in the same manner, reducing the chances of discrepancies between environments.
Speed: Automation speeds up the deployment process, allowing you to release new features and bug fixes faster.
Reliability: Automated tests can be run before deployment, catching issues early and ensuring that only stable code is deployed.
Scalability: Automation makes it easier to manage deployments across multiple environments and scale your applications as needed.
Besides that, automated continuous deployment frees up developers to focus on writing code rather than managing deployments. This can lead to increased productivity and a more enjoyable development experience. Learn more about the impact of Kubernetes on software release velocity.
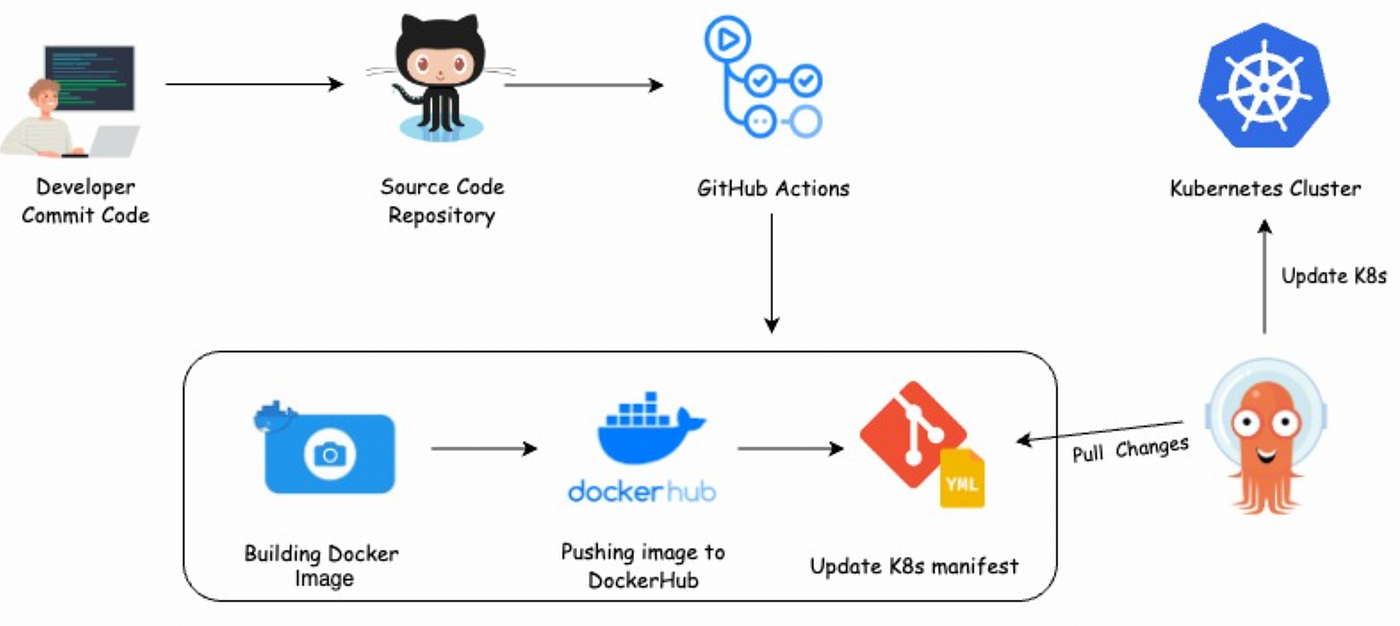
“CI/CD with GitHub, GitHub Actions, Argo …” from medium.com and used with no modifications.
Prerequisites and Setup
Before you can integrate GitHub Actions with Kubernetes, you’ll need to set up a few prerequisites. These include creating a GitHub repository, setting up a Kubernetes cluster, and configuring the necessary tools.
Setting Up Your GitHub Repository
The first step is to create a GitHub repository for your project. This repository will serve as the central location for your code and the GitHub Actions workflows that automate your CI/CD pipeline.
Here’s how to set up a GitHub repository:
Go to GitHub and log in to your account.
Click the “+” icon in the top right corner and select “New repository.”
Enter a name for your repository and provide a brief description.
Choose the repository’s visibility (public or private).
Click “Create repository.”
Once your repository is set up, you can start adding your code and creating GitHub Actions workflows to automate your CI/CD pipeline.
Creating a Kubernetes Cluster
The next step is to set up a Kubernetes cluster where your applications will be deployed. There are several ways to create a Kubernetes cluster, depending on your needs and the resources available to you.
Some popular options for creating a Kubernetes cluster include:
Minikube: A local Kubernetes cluster for development and testing purposes.
Google Kubernetes Engine (GKE): A managed Kubernetes service provided by Google Cloud Platform.
Amazon Elastic Kubernetes Service (EKS): A managed Kubernetes service provided by AWS.
Azure Kubernetes Service (AKS): A managed Kubernetes service provided by Microsoft Azure.
For this article, we’ll use Minikube to create a local Kubernetes cluster. Here’s how to do it:
Install Minikube by following the instructions on the Minikube website.
Start Minikube by running the following command in your terminal:
minikube start
Verify that Minikube is running by executing:
kubectl get nodes
With Minikube running, you now have a local Kubernetes cluster ready for deploying your applications.
Necessary Tools and Configurations
To integrate GitHub Actions with Kubernetes, you’ll need a few additional tools and configurations:
Docker: Docker is used to build container images for your applications. Install Docker by following the instructions on the Docker website.
kubectl: The Kubernetes command-line tool for interacting with your cluster. Install kubectl by following the instructions on the Kubernetes website.
GitHub Actions Secrets: Store sensitive information like API keys and access tokens securely in your GitHub repository. You can add secrets by navigating to your repository settings and selecting “Secrets.”
With these tools and configurations in place, you’re ready to start building your CI/CD pipeline with GitHub Actions.
Building Your CI/CD Pipeline with GitHub Actions
Now that you have your GitHub repository and Kubernetes cluster set up, it’s time to build your CI/CD pipeline using GitHub Actions. This involves creating workflows that automate the process of building, testing, and deploying your applications.
Writing Your First GitHub Actions Workflow
To get started, you’ll need to create a workflow file in your GitHub repository. This file defines the steps that GitHub Actions will take to build, test, and deploy your application.
Here’s an example of a simple GitHub Actions workflow file:
name: CI/CD Pipeline on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Set up Docker Buildx uses: docker/setup-buildx-action@v1 - name: Build and push Docker image uses: docker/build-push-action@v2 with: push: true tags: user/repository:latest - name: Deploy to Kubernetes uses: azure/k8s-deploy@v1 with: manifests: | ./k8s/deployment.yaml images: user/repository:latest
This workflow is triggered whenever you push changes to the main branch of your repository. It checks out your code, sets up Docker Buildx, builds and pushes a Docker image, and deploys the image to your Kubernetes cluster.
Writing Your First GitHub Actions Workflow
To get started, you’ll need to create a workflow file in your GitHub repository. This file defines the steps that GitHub Actions will take to build, test, and deploy your application.
Here’s an example of a simple GitHub Actions workflow file:
name: CI/CD Pipeline on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Set up Docker Buildx uses: docker/setup-buildx-action@v1 - name: Build and push Docker image uses: docker/build-push-action@v2 with: push: true tags: user/repository:latest - name: Deploy to Kubernetes uses: azure/k8s-deploy@v1 with: manifests: | ./k8s/deployment.yaml images: user/repository:latest
This workflow is triggered whenever you push changes to the main branch of your repository. It checks out your code, sets up Docker Buildx, builds and pushes a Docker image, and deploys the image to your Kubernetes cluster.
Automating Builds with Docker
Automating the build process with Docker is crucial for creating a seamless CI/CD pipeline. Docker allows you to package your application and its dependencies into a container, ensuring that it runs consistently across different environments.
In the workflow example above, the step that uses docker/build-push-action@v2
automates the process of building and pushing the Docker image. Here’s a breakdown of what this step does:
Builds the Docker image: Based on the Dockerfile in your repository, this action builds the Docker image.
Tags the Docker image: The image is tagged with the specified tags (e.g.,
user/repository:latest
).Pushes the Docker image: The built image is pushed to a Docker registry, making it available for deployment.
By automating the build process, you ensure that every time you push changes to your repository, a new Docker image is created and pushed, ready for deployment.
Continuous Testing Strategies
Testing is a critical part of any CI/CD pipeline. Continuous testing ensures that your application remains stable and functional as new changes are introduced. In GitHub Actions, you can add steps to your workflow to run automated tests.
Here’s an example of how you can integrate testing into your GitHub Actions workflow:
name: CI/CD Pipeline on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Set up Node.js uses: actions/setup-node@v2 with: node-version: '14' - name: Install dependencies run: npm install - name: Run tests run: npm test - name: Build and push Docker image uses: docker/build-push-action@v2 with: push: true tags: user/repository:latest - name: Deploy to Kubernetes uses: azure/k8s-deploy@v1 with: manifests: | ./k8s/deployment.yaml images: user/repository:latest
In this example, the workflow includes steps to set up Node.js, install dependencies, and run tests before building and pushing the Docker image. This ensures that only code that passes the tests is deployed.
Deploying to Kubernetes
Once your application is built and tested, the next step is to deploy it to your Kubernetes cluster. This involves configuring Kubernetes deployment manifests and integrating them with your GitHub Actions workflow.
Configuring Kubernetes Deployment Manifests
A Kubernetes deployment manifest is a YAML file that describes the desired state of your application in the Kubernetes cluster. This includes information about the Docker image to use, the number of replicas, and other configuration details.
Here’s an example of a simple Kubernetes deployment manifest:
apiVersion: apps/v1 kind: Deployment metadata: name: my-app spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-app image: user/repository:latest ports: - containerPort: 80
This manifest defines a deployment with three replicas of the my-app
container, using the Docker image user/repository:latest
. You can customize this manifest to suit the needs of your application.
Integrating Kubernetes with GitHub Actions
To deploy your application to Kubernetes using GitHub Actions, you need to add a step in your workflow that uses a Kubernetes deployment action. In the example workflow provided earlier, the step that uses azure/k8s-deploy@v1
handles the deployment.
This step requires the following inputs:
manifests: The path to your Kubernetes deployment manifest file.
images: The Docker image to deploy.
By including this step in your workflow, you ensure that your application is automatically deployed to your Kubernetes cluster whenever you push changes to your repository.
Managing Secrets and Environment Variables
Managing secrets and environment variables is crucial for the security and functionality of your deployment. GitHub Actions provides a secure way to store and access secrets, such as API keys and access tokens.
Here’s how to add secrets to your GitHub repository:
Navigate to your repository settings.
Select “Secrets” from the sidebar menu.
Click “New repository secret.”
Enter a name and value for the secret, then click “Add secret.”
In your workflow file, you can access these secrets using the secrets
context. For example:
jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Deploy to Kubernetes uses: azure/k8s-deploy@v1 with: manifests: | ./k8s/deployment.yaml images: user/repository:latest secret-name: ${{ secrets.K8S_SECRET }}
By securely managing secrets and environment variables, you ensure that your deployment process remains secure and efficient. For more insights, read about Kubernetes cluster performance tuning.
Case Study 1: E-Commerce Application
Imagine an e-commerce company that needs to deploy updates to its website multiple times a day. Before integrating GitHub Actions with Kubernetes, the team had to manually build, test, and deploy each update. This process was time-consuming and prone to errors. By integrating GitHub Actions with Kubernetes, the team automated their CI/CD pipeline, significantly reducing deployment times and improving reliability.
Now, every time a developer pushes code to the main branch, GitHub Actions automatically builds the Docker image, runs tests, and deploys the application to the Kubernetes cluster. This streamlined process has allowed the company to release new features and bug fixes more rapidly, enhancing the customer experience and keeping the business competitive.
Case Study 2: Financial Services Platform
A financial services platform with strict regulatory requirements needed a reliable and secure way to deploy updates. The manual deployment process was not only slow but also introduced potential security risks. By integrating GitHub Actions with Kubernetes, the platform’s development team was able to automate their CI/CD pipeline, ensuring consistent and secure deployments.
The team used GitHub Actions to automate the build and test processes, and Kubernetes to manage the deployment of containerized applications. They also leveraged GitHub Secrets to securely manage sensitive information, such as API keys and database credentials. As a result, the platform now enjoys faster deployment times, reduced risk of human error, and enhanced security, all while complying with regulatory requirements.
Final Thoughts
Integrating GitHub Actions with Kubernetes can revolutionize your DevOps processes, making continuous deployment more efficient, reliable, and secure. By automating the build, test, and deployment steps, you can reduce the risk of human error, speed up release cycles, and ensure consistent deployments across environments. Whether you’re working on a small project or managing a large-scale application, this integration can help you achieve a more streamlined and effective CI/CD pipeline.
Key Takeaways from Integrating GitHub Actions and Kubernetes
Integrating GitHub Actions with Kubernetes offers several key benefits:
Automated workflows reduce manual steps and minimize the risk of errors.
Consistent deployments ensure that your application runs smoothly across different environments.
Faster release cycles allow you to deliver new features and bug fixes more quickly.
Secure management of secrets and environment variables enhances the security of your deployments.
Scalability and reliability are improved, making it easier to manage large-scale applications.
Future Trends in DevOps Automation
The future of DevOps automation is very exciting, with several trends emerging that could further streamline and enhance the CI/CD process:
AI and Machine Learning: AI and machine learning can be used to optimize CI/CD pipelines, predict potential issues, and recommend improvements.
Serverless Architectures: Serverless computing can simplify deployment processes and reduce infrastructure management overhead.
Advanced Monitoring and Analytics: Enhanced monitoring and analytics tools can provide deeper insights into the performance and health of your applications.
Integration with More Tools: As the DevOps ecosystem continues to grow, integrating CI/CD pipelines with a wider range of tools and services will become more seamless.
Frequently Asked Questions (FAQ)
What are the primary advantages of using GitHub Actions with Kubernetes?
The primary advantages include automated workflows that reduce manual steps, consistent deployments across environments, faster release cycles, secure management of secrets, and improved scalability and reliability for managing large-scale applications.
How difficult is it to set up a CI/CD pipeline with GitHub Actions?
Setting up a CI/CD pipeline with GitHub Actions is relatively straightforward, especially if you follow the step-by-step instructions provided in this article. The process involves creating a GitHub repository, setting up a Kubernetes cluster, and configuring the necessary workflows and secrets.
Once these steps are completed, you can automate the build, test, and deployment processes, significantly simplifying your DevOps workflow.
Can I use GitHub Actions with other CI/CD tools?
Yes, GitHub Actions can be used in conjunction with other CI/CD tools to create a more comprehensive and customized pipeline. For example, you can integrate GitHub Actions with Jenkins, CircleCI, or Travis CI to leverage the strengths of each tool and create a more robust CI/CD pipeline.
By combining different tools, you can tailor your DevOps processes to meet the specific needs of your project and team.